返回目录:word文档
利用easy poi 实现word批量导出
easy poi 引入
实现功能
代码
easy poi 引入
maven
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-base</artifactId>
<version>3.2.0</version>
</dependency>
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-web</artifactId>
<version>3.2.0</version>
</dependency>
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-annotation</artifactId>
<version>3.2.0</version>
</dependency>
easy poi 作者官方文档
实现功能
实现基于word模板批量生成word文档,并压缩成压缩包进行导出;
代码
public void exportWord(String projectId, HttpServletResponse response) throws Exception{
//获取项目根目录
File path = new File(ResourceUtils.getURL("classpath:").getPath());
//导出前的检查
beforeExportWord(path);
//业务代码
//根据项目id获取项目信息
ProjectEntity entity = projectRepository.getOne(projectId);
response.setCharacterEncoding("UTF-8");
response.setContentType("application/x-download");
//把项目名称当作zip包的名字
String fileName = entity.getProjectName()+".zip";
fileName = URLEncoder.encode(fileName, "UTF-8");
response.addHeader("Content-Disposition", "attachment;filename=" + fileName);
//获取该项目下评测机构的list
List<PgzjEntity> pgzjList = pgzjRepository.findByProjectIdOrderByPgzjIdAsc(projectId);
List<PgzjCompanyEntity> companList = new ArrayList<>();
if(pgzjList.size()>0){
for(PgzjEntity pgzjItem: pgzjList){
PgjgInfoUserEntity pgjgInfoUser = pgjgInfoUserRepository.findByPgjgLoginIdAndProjectId(pgzjItem.getLoginId(), pgzjItem.getProjectId()).get(0);
PgjgInfoEntity pgjgInfo = pgjgInfoRepository.getOne(pgjgInfoUser.getPgjgInfoId());
//获取每个机构下评测的产品
companList = pgzjCompanyRepository.findByPgzjId(pgzjItem.getPgzjId());
if(companList.size()>0){
for(PgzjCompanyEntity companItem:companList){
//异步执行生成word方法
asyncService.caretWord(companItem,pgjgInfo,path,pgzjItem);
//生成的word文档放到word文件夹下
}
}
}
}
//业务代码结束
//超两分钟跳出循环,执行打,防止无限循环
Long startId = System.currentTimeMillis();
while (System.currentTimeMillis()-startId<120*1000){
int a = 0;
File word = new File(path.getAbsolutePath(), "/word");
System.out.println("开始:");
File[] arr = word.listFiles();
if(arr.length==(pgzjList.size()*companList.size())){
break;
}
System.out.println("等待:"+arr.length);
}
exportZip(path,response);
}
public void beforeExportWord(File path) throws Exception {
//获取临时存放生成word文件夹
File word = new File(path.getAbsolutePath(), "/word");
//判断文件夹是否存在,存在清空文件夹,不存在创建文件夹
if (word.exists()) {
for (File f : word.listFiles()) {
f.delete();
}
} else {
word.mkdir();
}
//获取临时存放生成zip文件夹
File zip = new File(path.getAbsolutePath(), "/zip");
//判断文件夹是否存在,存在清空文件夹,不存在创建文件夹
if (zip.exists()) {
for (File f : zip.listFiles()) {
f.delete();
}
} else {
zip.mkdir();
}
}
public void exportZip(File path,HttpServletResponse response) throws Exception{
FileOutputStream fos1 = new FileOutputStream(new File(path.getAbsolutePath()+"/zip/模板.zip"));
ZipUtils.toZip(path.getAbsolutePath()+"/word", fos1, true);
OutputStream fos = null;
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
fos = response.getOutputStream();
bis = new BufferedInputStream(new FileInputStream(path.getAbsolutePath()+"/zip/模板.zip"));
bos = new BufferedOutputStream(fos);
byte[] buff = new byte[2048];
int bytesRead;
while (-1 != (bytesRead = bis.read(buff, 0, buff.length))) {
bos.write(buff, 0, bytesRead);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
fos.flush();
bis.close();
bos.close();
fos.close();
}
}
}
public class ZipUtils {
private static final int BUFFER_SIZE = 2 * 1024;
public static void toZip(String srcDir, OutputStream out, boolean KeepDirStructure)
throws RuntimeException {
long start = System.currentTimeMillis();
ZipOutputStream zos = null;
try {
zos = new ZipOutputStream(out);
File sourceFile = new File(srcDir);
compress(sourceFile, zos, sourceFile.getName(), KeepDirStructure);
long end = System.currentTimeMillis();
System.out.println("压缩完成,耗时:" + (end - start) + " ms");
} catch (Exception e) {
throw new RuntimeException("zip error from ZipUtils", e);
} finally {
if (zos != null) {
try {
zos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
/**
* 压缩成ZIP 方法2
*
* @param srcFiles 需要压缩的文件列表
* @param out 压缩文件输出流
* @throws RuntimeException 压缩失败会抛出运行时异常
*/
public static void toZip(List<File> srcFiles, OutputStream out) throws RuntimeException {
long start = System.currentTimeMillis();
ZipOutputStream zos = null;
try {
zos = new ZipOutputStream(out);
for (File srcFile : srcFiles) {
byte[] buf = new byte[BUFFER_SIZE];
zos.putNextEntry(new ZipEntry(srcFile.getName()));
int len;
FileInputStream in = new FileInputStream(srcFile);
while ((len = in.read(buf)) != -1) {
zos.write(buf, 0, len);
}
zos.closeEntry();
in.close();
}
long end = System.currentTimeMillis();
System.out.println("压缩完成,耗时:" + (end - start) + " ms");
} catch (Exception e) {
throw new RuntimeException("zip error from ZipUtils", e);
} finally {
if (zos != null) {
try {
zos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
/**
* 递归压缩方法
*
* @param sourceFile 源文件
* @param zos zip输出流
* @param name 压缩后的名称
* @param KeepDirStructure 是否保留原来的目录结构,true:保留目录结构;
* false:所有文件跑到压缩包根目录下(注意:不保留目录结构可能会出现同名文件,会压缩失败)
* @throws Exception 101
*/
private static void compress(File sourceFile, ZipOutputStream zos, String name,
boolean KeepDirStructure) throws Exception {
byte[] buf = new byte[BUFFER_SIZE];
if (sourceFile.isFile()) {
// 向zip输出流中添加一个zip实体,构造器中name为zip实体的文件的名字
zos.putNextEntry(new ZipEntry(name));
// copy文件到zip输出流中
int len;
FileInputStream in = new FileInputStream(sourceFile);
while ((len = in.read(buf)) != -1) {
zos.write(buf, 0, len);
}
// Complete the entry
zos.closeEntry();
in.close();
} else {
File[] listFiles = sourceFile.listFiles();
if (listFiles == null || listFiles.length == 0) {
// 需要保留原来的文件结构时,需要对空文件夹进行处理
if (KeepDirStructure) {
// 空文件夹的处理
zos.putNextEntry(new ZipEntry(name + "/"));
// 没有文件,不需要文件的copy
zos.closeEntry();
}
} else {
for (File file : listFiles) {
// 判断是否需要保留原来的文件结构
if (KeepDirStructure) {
// 注意:file.getName()前面需要带上父文件夹的名字加一斜杠,
// 不然最后压缩包中就不能保留原来的文件结构,即:所有文件都跑到压缩包根目录下了
compress(file, zos, name + "/" + file.getName(), KeepDirStructure);
} else {
compress(file, zos, file.getName(), KeepDirStructure);
}
}
}
}
}
public static void main(String[] args) throws Exception {
/** 测试压缩方法1 */
FileOutputStream fos1 = new FileOutputStream(new File("E:\\\\IdeaProjects\\\\perfect\\\\perfect-consumer\\\\src\\\\main\\\\resources\\\\zip/mytest02.zip"));
ZipUtils.toZip("E:\\\\IdeaProjects\\\\perfect\\\\perfect-consumer\\\\src\\\\main\\\\resources\\\\word", fos1, true);
File file = new File("E:\\\\IdeaProjects\\\\perfect\\\\perfect-consumer\\\\src\\\\main\\\\resources\\\\zip");
if (file.isDirectory()) {
File[] files = file.listFiles();
for (File f : files) {
f.delete();
}
}
/** 测试压缩方法2 */
List<File> fileList = new ArrayList<>();
fileList.add(new File("D:/Java/jdk1.7.0_45_64bit/bin/jar.exe"));
fileList.add(new File("D:/Java/jdk1.7.0_45_64bit/bin/java.exe"));
FileOutputStream fos2 = new FileOutputStream(new File("c:/mytest02.zip"));
ZipUtils.toZip(fileList, fos2);
}
}
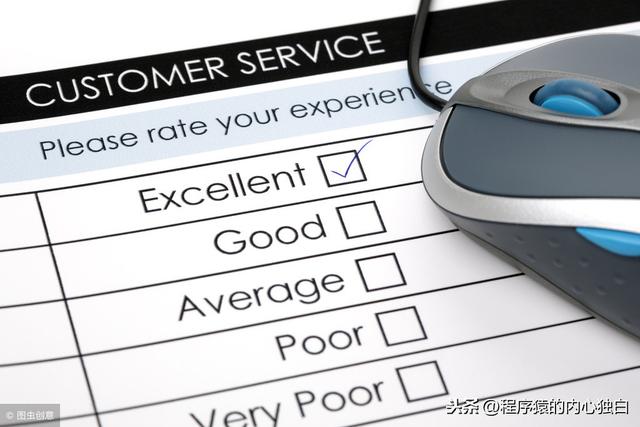